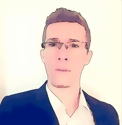
Python Function Parameters: The Essentials
For most intents and purposes, function parameters are a fairly basic concept. You define some inputs you want your function to take, and pass values for those parameters whenever you call the function.
Python's function parameter system is flexible and powerful, and going beyond the basic usage, I must say that with that power also comes some complexity. Even after all these years, I still sometimes find myself looking up certain constraints and syntax. I thought I'd summarize and break down the essentials here in a way that makes sense.
Note: for the rest of this article, we'll use both terms: function parameters and arguments. The general convention is that parameters are the input names/types in the function definition, whereas arguments are the actual values passed for these parameters in the function call. We'll respect this convention throughout the article.
Default arguments
Let's start with something simple like default arguments:
Nothing fancy here. If you don't pass a value, Python uses "Unknown". This is particularly useful for functions where most calls might use a common value, but you still want the flexibility to override it.
Positional vs keyword arguments
This distinction, and the confusion that comes with it, can stick for years until you realize it's not about how you define functions, but how you call them:
As shown in the example, it's the same function, we're just calling it differently.
Positional arguments depend on their position in the function call. The first value goes to the first parameter, second to the second, and so on. With keyword arguments, you explicitly say which parameter each value is for, which means you can pass them
in a different order.
But probably the biggest benefit of keyword arguments is that they make your code self-documenting and less error-prone. Compare these two calls:
In the first call, we don't know what those arguments after df mean. We'd have to hover over the function name, or go to its definition, to understand what's going on. That's of course quite easy to do with modern IDEs, but it does come with some cognitive burden.
The second call on the other hand, is clear enough right away. Granted, this does make your code more verbose, but sometimes it's a price worth paying, especially for functions that take many arguments, take boolean flags, or are a work in progress (relying on an ever-changing order can introduce subtle bugs).
You can mix both positional and keyword arguments in a single call, but there's a simple rule: positional arguments must come first, followed by keyword arguments.
Variable numbers of arguments
Sometimes you don't know in advance how many arguments a function might receive. Python has elegant solutions for both positional and keyword variable arguments.
Here's how you can pass arbitrary positional arguments (*args):
Inside the function, *args becomes a tuple containing all the positional arguments. You can have regular parameters before *args, but any parameter after it must be passed as a keyword argument.
Now let's see an example with arbitrary keyword arguments (**kwargs):
Here, **kwargs becomes a dictionary where the keys are the parameter names and the values are what you passed, and you can pass as many key-value pairs as you'd like.
Enforcing argument styles
Python 3 introduced syntax to control how arguments can be passed. This might seem like a bit too much, but it can be important when creating intuitive APIs/SDKs and preventing bugs.
Here's how you can enforce keyword-only arguments:
Everything after the *
must be passed as a keyword argument. This is a good idea for functions with multiple boolean flags or options that would be confusing if passed positionally.
Note: the * character itself is not an argument, but just a separator. It can even come first, in which case every argument must be passed as a keyword one.
Note 2: the parameters can be omitted if they have a default value. Default values work the same, no matter what styles or constraints we use.
Perhaps a more niche use case, but since Python 3.8, by using a forward slash (/
) you can mark parameters as positional-only well:
Parameters before the / must be passed positionally. This is useful when parameter names are implementation details that shouldn't be relied upon in calling code. One (better) example would be mathematical functions where the parameters have standard meanings (like pow(x, y)
).
Same as with the keyword arguments, a / at the start means all arguments must be passed positionally.
Everything, everywhere, all at once
You would rarely need to combine everything we talked about so far, in a single function definition/call. But it's interesting and useful to know how that would look like. Here's the general form of a Python function definition:
Where:
- pos_only_args
must be passed positionally
- normal_args
can be passed either way
- *args
captures excess positional arguments
- kw_only_args
must be passed as keywords
- **kwargs
captures excess keyword arguments
You can also disable variable mixing, with something like f(pos_only, /, *, kw_only)
. You still have both positional and mixed arguments in the same function call, but you determine which is which. So in this case, the caller client cannot pass kw_only positionally, and cannot pass pos_only by keyword.
So yeah, this can get a bit tricky if you go all out. The above should be a nice little cheatsheet for such cases.
The mutable default trap
This wouldn't be a complete article about function parameters in Python, without mentioning mutable defaults. It is probably the most notorious gotcha, and it can bite even senior developers. Thankfully IDEs like Pycharm now warn you when setting mutable defaults, but it's great to know why and how to avoid it.
Here's a simple example:
Our intuition tells us to expect the second call to return just ["banana"], but that's not how Python works.
Here's what's happening: default parameter values are evaluated only once, when the function is defined, not each time it's called. So that empty list [] is created exactly once when Python first encounters the function definition.
Every subsequent call to add_item without an items parameter uses the same list object from the first call. This is why the second call returns both "apple" and "banana"; we're still using and modifying the original list.
This behavior is especially cunning because it can lurk undetected in code that works perfectly during testing but fails mysteriously in production. The solution is to never use mutable objects (lists, dictionaries, sets, etc.) as default values. Instead, use this pattern:
Now each call gets its own clean list when you don't specify the items parameter.
Performance
It's worth mentioning that positional arguments are slightly faster than keyword arguments. That's because Python doesn't have to match names at runtime; it just uses the position.
The difference is negligible for most code, but in tight loops processing millions of items, it might matter and it's something to keep in mind.
Overview
Let's have a final summary of when to use what:
- Default parameters: when a parameter has a common value that makes sense most of the time.
- Keyword arguments: for readability, especially with boolean flags or when the meaning isn't obvious from the value.
- Positional-only: for functions where the parameters have standard meanings.
- Keyword-only: to force explicit parameter naming for clarity and prevent errors.
- *args: when the function can work with any number of inputs (like `max()`).
- **kwargs: when you want to accept arbitrary configuration options.
- Mutable defaults: never. Instead, use the common pattern of creating a new object if it was not passed.
Understanding these options gives you all the flexibility you need to design clean, robust and backward-compatible function interfaces. Good parameter design is about making your code hard to use incorrectly and easy to read later. Sometimes that means restricting options, not adding them.