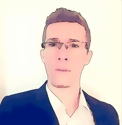
Bare return vs return None vs no return
Python is quite flexible regarding the usage of the return statement.
Unlike in languages like Java or C#, where you have to specify a return type in the function signature (void when it doesn't return anything), a function signature in Python is exempt from that. As of recently, you could use annotations to hint at the expected return type, but it has no effect on the interpreter.
Nevertheless, just like in other languages, there are functions which should return something, and functions which shouldn't. It depends entirely on the logic you're trying to implement. Python, and similar languages, simply allow you to be more 'creative' with the return statement.
If the function should return a value, the return statement in Python is no different than the languages I mentioned earlier:
But what if the function isn't supposed to return anything ? A great example are methods which act on the object at hand, such as my_list.sort()
. That'll simply sort the list object calling it.
And, what if the function isn't supposed to return anything for the actual input arguments ?
Every function in Python which does not hit a return statement, will implicitly return None.
This leaves the Python developer with a few options:
- don't do anything (the function will implicitly return None
)
- have an explicit return None
statement
- have a bare return statement
Now, all of these options will return None
, so there's no difference in behavior. It's purely a choice of coding style, but that doesn't mean it's irrelevant. A good style will make the code more expressive and readable.
Goes without saying that style is debatable at least to some extent, and there's no absolute right and wrong here. I'll just let you know how I personally go about it, and my short reasoning for it.
I go with the option of having a return None
, when the function is indeed meant to return a value, but it can't do so on this particular conditional path. That could be due to invalid input, exceptions, or whatever business logic requirement.
I'll use no return statement whatsoever when the function simply isn't meant to return. That's just keeping things clean. Any return statement in this case adds on to code clutter.
I'll use a bare return when the function isn't meant to return, and I want to exit the function early. Sort of using it as a break statement. It's not something I do often though, and probably a remnant of my Java days.